ASP.NET ile Gmail üzerinden mail gönderme
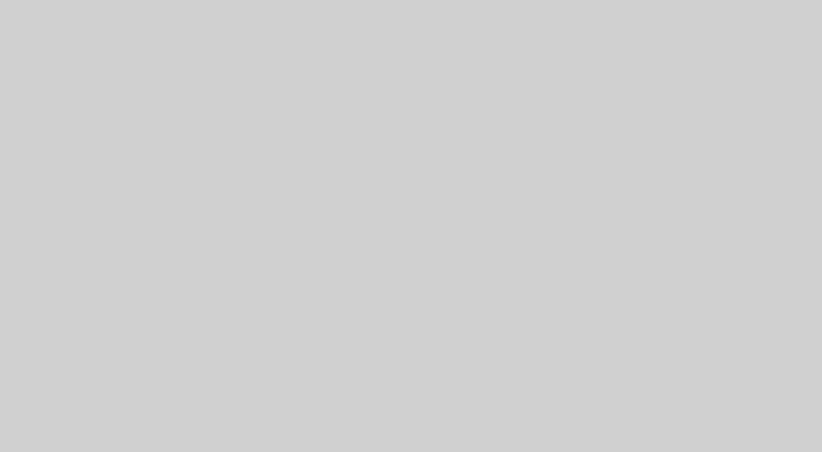
ASP.NET MVC ile Gmail üzerinden mail göndermek için aşağıdaki void kullanılabilir. Burada mail gönderen gmail hesabı deneme@gmail.com dır. deneme2@gmail.com ise mail gönderilen mail adresi bu adres dinamik olarak belirlenebilir.
MVC kullandığım için Model ve Control yapısı aşağıdaki gibidir. Ayrıca veri tabanı işlemini dapper kullanarak yaptım. Bu örnekte web sayfanızdaki iletişim formu doldurulduktan sonra size mesaj veri tabanına kayıt edildikten sonra ayrıca bilgi olarak mail adresinize mail gönderilmektedir.
Gmail ayarlarından bir uygulamanın mail göndermesine izin verilmek için aşağıdaki linkteki yazıyı okuyabilirsiniz.
GMail üzerinden mail gönderme İzni verme
Model
namespace Deneme.Models
{
public class Contact
{
public int Id { get; set; }
public Nullable<System.DateTime> Date { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
public string Subject { get; set; }
public string Message { get; set; }
}
}
Control
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using Deneme.Models;
using Dapper;
using System.Net.Mail;
namespace Deneme.Controllers
{
public class IletisimController : Controller
{
public ActionResult Index()
{
ViewBag.Error = 0;
return View();
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Index(Contact contact)
{
if (ModelState.IsValid)
{
using (var connection = DBContext.GetOpenConnection())
{
contact.Date = DateTime.Now;
var sqlQuery = @"INSERT INTO Contact(Name,EMail,Phone,Subject,Message,Date)
VALUES (@Name,@EMail,@Phone,@Subject,@Message,@Date)";
connection.Execute(sqlQuery, contact);
ViewBag.Error = 1;
this.MailGonder(contact);
}
return View(contact);
}
else
{
ViewBag.Error = 2;
}
return View();
}
public void MailGonder(Contact contact)
{
MailMessage loginInfo = new MailMessage();
if (contact.Email != null)
{
loginInfo.To.Add("deneme2@gmail.com");
loginInfo.From = new MailAddress("deneme@gmail.com");
loginInfo.Subject = contact.Subject;
loginInfo.Body = contact.Message + "<br/>" + "Mail Gönderenin Adı Soyadı:" + contact.Name + "<br/>" + "Mail Gönderenin Telefon Numarası:" + contact.Phone + "<br/>" + "Mail Gönderenin Mail Adresi:" + contact.Email;
loginInfo.IsBodyHtml = true;
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.Port = 587;
smtp.EnableSsl = true;
smtp.Credentials = new System.Net.NetworkCredential("deneme@gmail.com", "Şifre");
smtp.Send(loginInfo);
}
}
}
}